– React + Spring Boot + PostgreSQL: CRUD example. For more information see the official Spring Boot docs on how to Enable HTTPS When Running behind a Proxy Server. 03 Step 38 - Calling Hello World HTTP Service with Path Variables When you want to get a public resource from a different origin, the resource-providing server needs to tell the browser "This origin where the request is coming from can access my resource". This annotation makes the annotated methods/classes as permitting cross-origin Now, CORS may be easy, but if you do not pay attention it will still cause some silly errors that can make you loose an absurd amount of time due to some very uninformative message errors. The browser remembers that and allows cross-origin resource sharing. Cross-origin resource sharing (CORS) is a mechanism that controls the AJAX calls to your resources outside the current origin. How to Enable Spring Boot CORS Example: In this tutorial, we are going to see How to Enable Spring Boot CORS example. In the last post we tried securing our Spring MVC app using spring security Spring Boot Security Login Example.We protected our app against CSRF attack too. The remote service to which you are making your AJAX request does not accept cross origin AJAX requests from your domain. Browsers (without CORS) can't do cross-origin requests. Cross-Origin Resource Sharing (CORS) is a security concept that allows restricting the resources implemented in web browsers. Access-Control-Allow-Origin: specifies the authorized domains to make cross-domain request. 03 Step 37 - Calling Hello World Bean JSON API from React Frontend. Note that the scheme, host, and port of the values must match exactly. The above configuration enables the filter but does not relax the cross-origin policy. CORSflare is a reverse proxy written in JavaScript that can be used to bypass most common Cross-Origin Resource Sharing restrictions, such as the errors that prevent to embed an external web page within a IFRAME element:. Spring Boot @CrossOrigin Annotation Example. CORS is a protocol that defines how a client (the browser) and a server negociate allowing the handling of cross origin requests via HTTP headers. How to fix: Cross origin requests are only supported for protocol schemes Some time ago I wrote a post about how to enable CORS in a web API . more options. 1. For more information, go to the Cross-Origin Resource Sharing W3C Recommendation. returning nothing) on the cross-origin requests that they are willing to service. It is designed to prevent the browser from delivering certain cross-origin network responses to a web page, when they might contain sensitive information and are not needed for existing web features. It is the web client (wherever the web client that is blocked happens to be placed in your setup) that does the actual blocking, so you need to permit the source address the client is intending to use with the injected Access-Control-Allow-Origin header.. In the spring.datasource.url property, mem is the name of an in-memory database and testdb is the name of schema that H2 provides, by default. CORS defines a way for client web applications that are loaded in one domain to interact with resources in a different domain. To determine whether a header header is a CORS-safelisted request-header, run these steps: . The request's Origin header must match an AllowedOrigin element.. CORS (Cross-Origin Resource Sharing) is a way for the server to say “I will accept your request, even though you came from a different origin.”. Spring CORS example using @CrossOrigin – Spring Boot. With the introduction of CORS, domains A and B can now share resources with each other without being blocked by the browser. This annotation makes the annotated methods/classes as permitting cross-origin requests. Finally discovered (from stackoverflow) out how to send Cross Domain Request to Sugar API with the custom header oauth-token included. Download the resulting ZIP file, which is an archive of a web application that is … While making API calls from a browser app with cross-origin, we frequently get errors as Cross-Origin Request Blocked and the API call fails. Please try again. Those who often read this blog already know that we’re deeply in love with NGINX, a lightweight, high-performance and open-source web server and reverse proxy used by more than 358 million websites and over 66% of the world’s top 10,000 websites. Rate limiting is the process of controlling traffic to a server based on client IPs, blocked IPs, geolocation, and other factors. The CORS policy is enforced by the browser. Basically process of allowing other sites to call your Web API is called CORS. We can also define our own schema and database. My spring boot app is running on port 8080, and my front-end (React.js) is running on port 3000. Cross-Origin Resource Sharing (CORS) can define a way in which MOTECH-UI and MOTECH-CORE interact to determine safely whether or not to allow the cross-origin request. In this context, "other origins" means the URL being accessed differs from the location that the JavaScript is running f… In such a case, CORS enables cross-domain communication. All the others. Enable for whole spring boot. Last modified: June 5, 2021 bezkoder React. example.com) is different from the host that serves the data (e.g. This extension enables server-side applications to enforce limitations (e.g. If the origin in a browser's request matches an origin in your CORS configuration, Cloud Storage returns Access-Control-Allow-Origin … You must make sure the user is logged in before you make the AJAX request to avoid being redirected. In this tutorial, I will show you how to build a Vue.js 2 CRUD Application to consume REST APIs, display and modify data using Vue 2, Vue Router and Axios. I want to add CORS support to my server. headers. In this quick tutorial, we'll set up a similar CORS configuration using Spring's 5 WebFlux framework. Enable the following CORS headers on the server. In this tutorial, I will show you how to build a React.js CRUD Application to consume Web API, display and modify data with Router, Axios & Bootstrap. if you’re using an external API), this approach won’t work. This can be resolved if … I'm also having the same problem right now. a web page on any domain - can make requests to your API. There is a mechanism that allows you to communicate to the browser which assets can be loaded at specific origins safely. In short, it say that the request are not intended to be used from the frontend. A cross-origin request is a request for website resources external to the origin. To overcome this, we have something called Cross Origin Resource Sharing (CORS). More Practice: – Vue.js JWT Authentication with Vuex and Vue Router – Vue File Upload example using Axios Fullstack: – Vue.js + Node.js + Express […] build your own proxy. The request's Origin header must match an AllowedOrigin element.. Spring 4.2+ provides build in support to for the Cross-origin … The problem is that my front-end does not get blocked by cors policy. During a redirect, the Origin is set to 'null', and the final destination of your request will then reject the request because you're not allowed to make cross-origin AJAX requests. Cross-Origin-Resource-Sharing (CORS) is a W3C specification which defines how a browser should be allowed using script to access different origin than the origin it has been served. The CORS policy is enforced by the browser. I also agree with a comment on a similar issue #4930 (comment). fonts, CSS or static images from CDN.CORS helps in serving web content from multiple domains into browsers who usually have the same-origin security policy.. If session expired means in the console it is trying to redirect to login page but the cross-origin request is blocking . CORS defines a way for client web applications that are loaded in one domain to interact with resources in a different domain. Access-Control-Allow-Credentials: specifies if cross-domain requests can have authorization credentials or not. CORS instructs the browser to determine if a cross-origin request (such as an image or JavaScript from b.secondexample.com) is allowed by a.example.com. Only headers … Browsers prevent Ajax requests receiving a response from a server that's on a different domain from the page that made the request. Fullstack: – React + Spring Boot + MySQL: CRUD example. set ("Origin", new URL (apiUrl). The browser remembers that and allows cross-origin resource sharing. Every header listed in the request's Access-Control-Request-Headers header on the preflight request must match an AllowedHeader element. springboot-websocket (backend): This project is used to develop WebSocket endpoint at the server-side using spring boot, STOMP, and sock js support. For example, a.example.com attempts to serve resources from b.secondexample.com. The reason why your example works when using fetch is because those options are part of the Request API (docs for mode are here ). For a detailed description, see Cross-Origin Resource Sharing (CORS) - HTTP | MDN. suganthi. Persons with disabilities may request reasonable modifications to access or participate in DNR programs and services by contacting the DNR ADA Title II coordinator at info.dnr@state.mn.us or 651-259-5488. Spring 5. Cross-Site Request Forgery Prevention Cheat Sheet¶ Introduction¶. Before CORS, JSONP was used to circumvent this restriction. Access to fetch at ' api end point' from origin ' https://webapp.io' has been blocked by CORS policy: Response to preflight request doesn ' t pass access control check: No ' Access-Control-Allow-Origin ' header is present on the requested resource. To specify what kind of cross-domain requests are authorised in our app, we are going to configure CORS globally. To configure Web Origin in Keycloak, you need to go to the Client’s configuration section. Cross-Origin Read Blocking (CORB) is a new web platform security feature that helps mitigate the threat of side-channel attacks (including Spectre). Spring Security and CORS. Disable CRSF (Cross-Site Request … Click Dependencies and select Rest Repositories and Spring Data Neo4j. Configuring CORS w/ Dynamic Origin. If you call the cors middleware in your Express application without passing any configuration options, by default it will add the CORS response header Access-Control-Allow-Origin: * to your API's responses. The cross-origin resource standard is used ONLY to enable browsers to issue cross-origin XHR, media, script, stylesheet, and WebGL texture HTTP requests then grant access to that data to other domains. Finally, we're ready to run our application. The browser will not allow you to get the sensitive data from other domain, for security purposes your browser will return to you “No ‘Access-Control-Allow-Origin'”. This is a bit worrying, because I want other origins to be blocked by cors policy when I deploy the app at a later point in time. This module supports validating the origin dynamically using a function provided to the origin option. It allows the clients to communicate servers from different origin. Once the Spring Boot application has been started, let's open a command console and type the following command: ng serve --open. The CORS specification identifies a collection of protocol headers of which Access-Control-Allow-Origin is the most significant. For security reasons, web browsers will prevent JavaScript code from making requests to a different domain (also known as the origin) than the one it's hosted on. Please use @CrossOrigin on the backend side in Java Spring boot controller (either class level or method level) as the solution for Chrome error'No 'Access-Control-Allow-Origin' header is present on the requested resource.' Union Pacific Corporation Announces 10% Dividend Increase for Second Quarter 2021 05/13/21 Union Pacific Honors Safe Chemical Transporters with … Cross-Origin Resource Sharing (CORS) is a mechanism that browsers and webviews — like the ones powering Capacitor and Cordova — use to restrict HTTP and HTTPS requests made from scripts to resources in a different origin for security reasons, mainly to protect your user's data and prevent attacks that would compromise your app. In this example, we will learn to enable Spring CORS support in Spring MVC application at method level … The SNA is best accessed from an app such as the free Avenza app Jodrell Ave. in Linwood. By CORS, communications between the same domain will be allowed to users and the communications that are cross-originated will be restricted to a few techniques. The File service supports CORS beginning with version 2015-02-21. Spring Rest Service allow Cross Origin - Java Developer Zone Remember, the same-origin policy tells the browser to block cross-origin requests. Now a days all the latest browsers are developed to support Cross Origin Request Security (CORS), however sometimes CORS still creates problem and it happens due to Java script or Ajax requested from another domain. Boot Lake SNA is open for bird and wildlife The request method (for example, GET or PUT) or the Access-Control-Request-Method header in case of a preflight OPTIONS request must be one of the AllowedMethod elements. With CORS, we can specify what kind of cross domain requests are authorized in a flexible way, instead of using some less secured and less … The rest of this post (Part 1) will show in detail how to implement a Spring Boot-based REST API for an Angular 2 app, and how to implement CORS so as to allow the Angular UI application to work with the REST API served from a different port and/or a different domain. I deployed Spring Boot app as backend and Angular app as frontend to heroku and faced CORS restrictions. I have been able to successfully implement user logging in but I am facing difficulty in logging the user out. The gateway can be configured to control CORS behavior. However, sometimes you might want to let other sites call your web API. This bridge allows Spring Boot projects to use the normal configuration toolchain while letting them configure the Netflix tools as documented (for the most part).
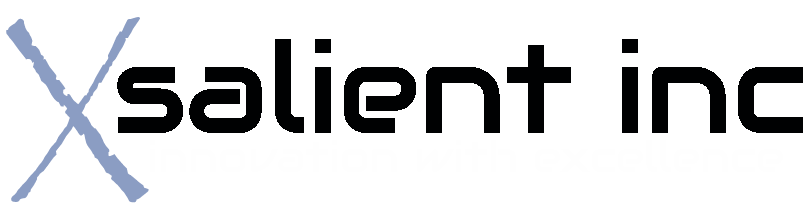
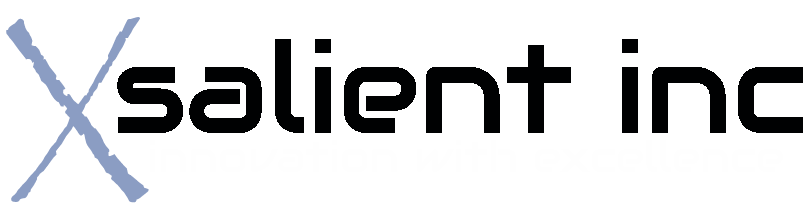